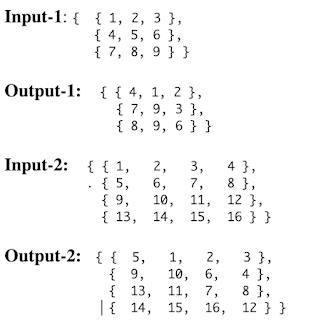
Rotate matrix by clockwise
Given a NxN matrix and need to rotate by clockwise as given below
Examples:
Let's see simple java code to rotate given NxN matrix.
OUTPUT:
Examples:
public class MatrixRotate { private int prev, curr; public static void main(String[] args) { int[][] matrix = new int[][] { { 1, 2, 3, 4 }, { 5, 6, 7, 8 }, { 9, 10, 11, 12 }, { 13, 14, 15, 16 } }; MatrixRotate obj = new MatrixRotate(); obj.rotateMatrix(matrix); for (int i = 0; i < matrix.length; i++) { System.out.println(); for (int j = 0; j < matrix[0].length; j++) { System.out.print(matrix[i][j] + ", "); } } } public void rotateMatrix(int[][] matrix) { int r = matrix.length; int c = matrix[0].length; int row = 0, col = 0; for (int cnt = 0;cnt<(r*c);cnt++) { prev = matrix[row + 1][col]; for (int i = col; i < c; i++) { swap(row, i, matrix); cnt++; } row++; for (int i = row; i < r; i++) { swap(i, c - 1, matrix); cnt++; } c--; if (row < r) { for (int i = c - 1; i >= col; i--) { swap(r - 1, i, matrix); cnt++; } } r--; if (col < c) { for (int i = r - 1; i >= row; i--) { swap(i, col, matrix); cnt++; } } col++; } } public void swap(int i, int j, int matrix[][]) { curr = matrix[i][j]; matrix[i][j] = prev; prev = curr; } }
OUTPUT:
5, 1, 2, 3, 9, 10, 6, 4, 13, 11, 7, 8, 14, 15, 16, 12,