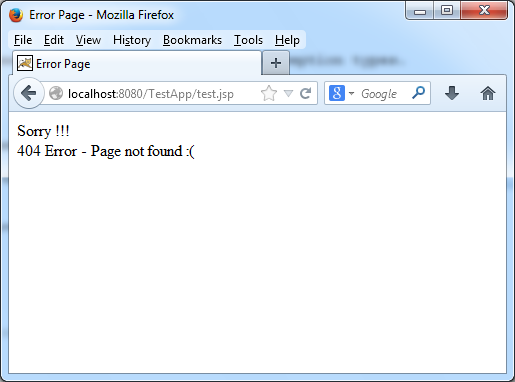
Creating custom error pages and handling exceptions in JSP
We all know how to handle exception in java code. But if there are any exception occurred at client side jsp files then how we can handle?
Basically Exception is an Object thrown at compile or run-time. When we talk about web application and handling exceptions in JSP files then there are 2 ways to handle it
1. By adding <error-page> element in web.xml for different error codes and exceptions.
2. By errorPage and isErrorPage attributes in page directives.
First lets see simple example by adding <error-page> element in web.xml for handling error codes.
1. Install Eclipse IDE and Apache Tomcat
2. Create Dynamic Web Application in Eclipse IDE and add these files
Folder Structure:
TestApp
|-> WEB-INF
|-> web.xml
|-> error404.jsp
|-> expError.jsp
|-> test_exception.jsp
Basically Exception is an Object thrown at compile or run-time. When we talk about web application and handling exceptions in JSP files then there are 2 ways to handle it
1. By adding <error-page> element in web.xml for different error codes and exceptions.
2. By errorPage and isErrorPage attributes in page directives.
First lets see simple example by adding <error-page> element in web.xml for handling error codes.
1. Install Eclipse IDE and Apache Tomcat
2. Create Dynamic Web Application in Eclipse IDE and add these files
- web.xml
- error404.jsp
- expError.jsp
- test_exception.jsp
Folder Structure:
TestApp
|-> WEB-INF
|-> web.xml
|-> error404.jsp
|-> expError.jsp
|-> test_exception.jsp
web.xml:
<?xml version="1.0" encoding="ISO-8859-1"?>
<web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
version="3.0">
<display-name>Error Code Test Application</display-name>
<description>Test Application for Error codes and Exceptions</description>
<error-page>
<error-code>404</error-code>
<location>/error404.jsp</location>
</error-page>
<error-page>
<error-code>500</error-code>
<location>/error500.jsp</location>
</error-page>
<error-page>
<exception-type>java.lang.Throwable</exception-type>
<location>/expError.jsp</location>
</error-page>
</web-app>
Here we have configured <error-page> elements for 404 error page and exception types.
error404.jsp:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Error Page</title>
</head>
<body>
Sorry !!!
<br> 404 Error - Page not found :(
</body>
</html>
If user tries to open page which not found then automatically error404.jsp page will be called. We will try to access page which not present in the path like test.jsp.
expError.jsp:
<%@page import="java.io.PrintWriter"%>
<%@page import="java.io.StringWriter"%>
<%@ page isErrorPage="true"%>
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Error Page</title>
</head>
<body>
Sorry !!!
<br>
<B>Exception occurred : </B><%=exception.getMessage()%>
<br>
<b>StackTrace: </b>
<%
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
exception.printStackTrace(pw);
out.println(sw);
pw.close();
sw.close();
%>
</body>
</html>
Next we will test for exceptions in Jsp file. expError.jsp file is a generic file to handle all types of run-time exceptions based on <exception-type> element in web.xml. Here we printing exception type and its stack trace.
test_exception.jsp:
test_exception.jsp page will be used to test exceptions. Here we are generating divide by Zero exception which will automatically thrown to expError.jsp page.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Test Exception Page</title>
</head>
<body>
<%
int val = 100;
val = val / 0;
%>
</body>
</html>
2. By errorPage and isErrorPage attributes in page directives.
2nd type is by adding errorPage attribute of page directive in actual pages and isErrorPage attribute in error pages as like below.
<%@ page errorPage="expError.jsp" %>
<%@ page isErrorPage="true" %>
To test without web.xml, remove <error-page> elements from web.xml and just add <%@ page errorPage="expError.jsp" %> page directive in top of test_exception.jsp and test through browser which will redirect to expError.jsp page.