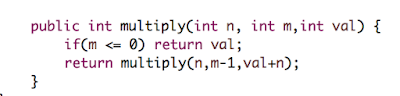
Multiply 2 numbers using recursion
In many interviews, interviewer may asked to write a simple program to multiply 2 integers without using '*' operator. We can solve this program by many ways like using looping, recursion, bitwise operator etc.,
In this tutorial we will see how to multiply 2 integers using recursion. As simple as method takes 1st (n) and 2nd (m) integer as argument and make recursive call m times. Each time summing with n and returning the final sum.
Lets see simple example to multiply 2 integers using recursion.
public class MultiplyNumber { public static void main(String[] args) { int val = new MultiplyNumber().multiply(7, 5); System.out.println(val); } public int multiply(int n, int m) { if(m>n) { int tmp = n; n = m; m = tmp; } return multiply(n,m,0); } public int multiply(int n, int m,int val) { if(m <= 0) return val; return multiply(n,m-1,val+n); } }
OUTPUT:
35