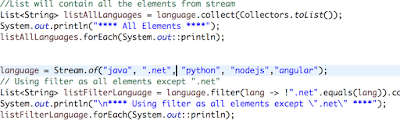
Java 8 - Stream and Filter
Stream API:
A stream gives a set of elements of specific type in a sequential order and it gets/computes elements on demand. But never stores the elements one the stream is been processed. Same thing we see in the below example.
Filter:
Applying filter on stream while computing and geting only the elements on specified conditions satisfied.
Before Java 8 if we need to filter some values on List, then only the option is to iterate on each element by External Iterator (for each loop). But in Java 8 we can use filter on top of stream to get those filtered elements from the given List. Lets see simple example as how to stream and then using filter on it to collect the final elements which we need from the list.
OUTPUT:
A stream gives a set of elements of specific type in a sequential order and it gets/computes elements on demand. But never stores the elements one the stream is been processed. Same thing we see in the below example.
Filter:
Applying filter on stream while computing and geting only the elements on specified conditions satisfied.
Before Java 8 if we need to filter some values on List, then only the option is to iterate on each element by External Iterator (for each loop). But in Java 8 we can use filter on top of stream to get those filtered elements from the given List. Lets see simple example as how to stream and then using filter on it to collect the final elements which we need from the list.
import java.util.List; import java.util.stream.Collectors; import java.util.stream.Stream; public class StreamFilter { public static void main(String[] args) { Stream<String> language = Stream.of("java", ".net", "python", "nodejs","angular"); //List will contain all the elements from stream List<String> listAllLanguages = language.collect(Collectors.toList()); System.out.println("**** All Elements ****"); listAllLanguages.forEach(System.out::println);
/* Here we can see stream getting empty and throwing IllegalStateException
* if we didn't recreate stream again. Since all the elements are streamed already
* and stream will be empty as of now.
*/ language = Stream.of("java", ".net", "python", "nodejs","angular"); // Using filter as all elements except ".net" List<String> listFilterLanguage = language.filter(lang -> !".net".equals(lang)).collect(Collectors.toList()); System.out.println("\n**** Using filter as all elements except \".net\" ****"); listFilterLanguage.forEach(System.out::println); } }
OUTPUT:
**** All Elements **** java .net python nodejs angular **** Using filter as all elements except ".net" **** java python nodejs angular