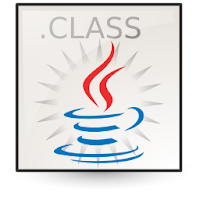
Difference between ClassNotFoundException and NoClassDefFoundError
When we see in general both ClassNotFoundException and NoClassDefFoundError are errors which comes when JVM or Class Loader not able to find appropriate class while loading at run-time. ClassNotFoundException is a checked exception and NoClassDefFoundError is an Error which comes under unchecked. There are different types of Class Loader loads classes from difference sources, sometimes it may cause library JAR files missing or incorrect class-path which causes loader not able to load the class at run-time. Even though both names sounds similar to class file missing there are difference between ClassNotFoundException and NoClassDefFoundError. |
ClassNotFoundException:
ClassNotFoundException comes when we try to load class at run-time using Reflection and if those class files are missing then application or program thrown with ClassNotFoundException Exception. There is nothing to check at compile time since its load those class at run-time. Best example is when we try to load JDBC driver class using reflection and we fail to point driver jars. Then program thrown with ClassNotFoundException as like below example.
import java.sql.Connection;
import java.sql.DriverManager;
public class ExceptionTest {
public static void main(String[] args) {
Connection conn = createConnection();
}
public static Connection createConnection() {
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
System.out.println("MySQL JDBC Driver Registered!");
conn = DriverManager.getConnection(
"jdbc:mysql://192.168.1.2:3306/mydatabase", "root", "root");
} catch (Exception e) {
System.err.println("Please configure mysql driver file !!!");
e.printStackTrace();
}
return conn;
}
}
OUTPUT:
Please configure mysql driver file !!!
java.lang.ClassNotFoundException: com.mysql.jdbc.Driver
at java.net.URLClassLoader$1.run(Unknown Source)
These are the different class loaders from difference classes like,
forName method in class Class.
loadClass method in class ClassLoader.
findSystemClass method in class ClassLoader.
NoClassDefFoundError:
NoClassDefFoundError is thrown when a class has been compiled with a specific class from the class path but if same class not available during run-time. Missing JAR files are the most basic reason to get NoClassDefFoundError. As per Java API docs "The searched-for class definition existed when the currently executing class was compiled, but the definition can no longer be found."
Lets see simple example to get NoClassDefFoundError Error.
public class SecondTestClass {
public SecondTestClass(){
System.out.println("Hello Java");
}
}
public class ErrorTest {
public static void main(String[] args) {
new SecondTestClass();
}
}
NOTE:
Once you create both java files compile SecondTestClass.java and ErrorTest.java classes separately. Next delete the generated class file called SecondTestClass.class. Next try to run ErrorTest.class file which will thrown with NoClassDefFoundError error message.
OUTPUT:
Exception in thread "main" java.lang.NoClassDefFoundError: SecondTestClass
at com.db.ErrorTest.main(ErrorTest.java:7)
Caused by: java.lang.ClassNotFoundException: SecondTestClass